Android Intent Tutorial
INTENT :
Android Intent is the message that is passed between components such as activities, content providers, broadcast receivers, services etc.
It is generally used with startActivity() method to invoke activity, broadcast receivers etc.
The dictionary meaning of intent is intention or purpose. So, it can be described as the intention to do action.
The LabeledIntent is the subclass of android.content.Intent class.
Android intents are mainly used to:
- Start the service
- Launch an activity
- Display a web page
- Display a list of contacts
- Broadcast a message
- Dial a phone call etc.
TYPES OF INTENT :
There are following two types of intents supported by Android
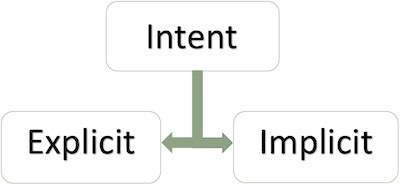
Explicit Intents :
Explicit intent going to be connected internal world of application,suppose if you wants to connect one activity to another activity, we can do this quote by explicit intent, below image is connecting first activity to second activity by clicking button.
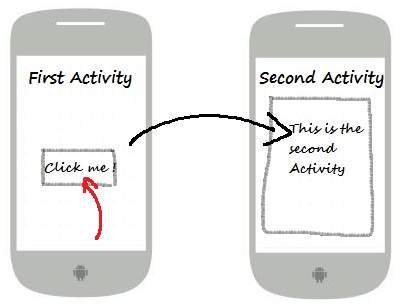
These intents designate the target component by its name and they are typically used for application-internal messages - such as an activity starting a subordinate service or launching a sister activity. For example −
// Explicit Intent by specifying its class name Intent i = new Intent(FirstActivity.this, SecondActivity.class); // Starts TargetActivity startActivity(i);
Implicit Intents
These intents do not name a target and the field for the component name is left blank. Implicit intents are often used to activate components in other applications. For example −
Intent read1=new Intent(); read1.setAction(android.content.Intent.ACTION_VIEW); read1.setData(ContactsContract.Contacts.CONTENT_URI); startActivity(read1);
INTENT EXAMPLE CODE
ANDROID MANIFEST FILE
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="intent.app.com.intent">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".SecondActivity"></activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="intent.app.com.intent">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".SecondActivity"></activity>
</application>
</manifest>
LAYOUT FILES
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="intent.app.com.intent.MainActivity">
<android.support.v7.widget.AppCompatEditText
android:id="@+id/name"
android:layout_marginTop="10dp"
android:imeOptions="actionNext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/enter_user_name"
/>
<android.support.v7.widget.AppCompatEditText
android:id="@+id/password"
android:imeOptions="actionGo"
android:layout_marginTop="10dp"
android:hint="@string/enter_password"
android:inputType="textPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<android.support.v7.widget.AppCompatButton
android:id="@+id/Submit"
android:text="Submit"
android:layout_marginTop="10dp"
android:background="@color/colorAccent"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
activity_second.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="intent.app.com.intent.SecondActivity"> <android.support.v7.widget.AppCompatTextView android:id="@+id/s_name" android:layout_marginTop="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/s_password" android:layout_marginTop="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" /> <android.support.v7.widget.AppCompatButton android:id="@+id/close" android:layout_marginTop="10dp" android:text="Close" android:layout_width="match_parent" android:background="@color/colorPrimaryDark" android:textColor="#FFFFFF" android:layout_height="wrap_content" /> </LinearLayout>
JAVA FILES
MainActivity.java
package intent.app.com.intent;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
// Initializing variables
EditText ename, epswd;
Button submit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ename = (EditText)findViewById(R.id.name);
epswd = (EditText)findViewById(R.id.password);
submit = (Button)findViewById(R.id.Submit);
//Listening to button event
submit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//Starting a new Intent
Intent intent= new Intent(MainActivity.this,SecondActivity.class);
//Sending data to another Activity
intent.putExtra("Uname",ename.getText().toString());
intent.putExtra("pswd",epswd.getText().toString());
startActivity(intent);
}
});
}
}
SecondActivity.java
package intent.app.com.intent; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class SecondActivity extends AppCompatActivity { Button close; TextView sname,spswd; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); sname = (TextView)findViewById(R.id.s_name); spswd = (TextView)findViewById(R.id.s_password); close = (Button)findViewById(R.id.close); //For get Intent in Second Screen Intent i = getIntent(); // Receiving the Data String Uname = i.getStringExtra("Uname"); String pswd = i.getStringExtra("pswd"); // Displaying Received data sname.setText(Uname); spswd.setText(pswd); // Binding Click event to Button close.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //Closing SecondScreen Activity finish(); } }); } }
Comments
Post a Comment